일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 정렬
- nestjs
- typeORM
- react
- TypeScript
- MongoDB
- Nest.js
- jest
- flask
- 게임
- MySQL
- game
- Python
- mongoose
- Bull
- cookie
- OCR
- Sequelize
- nodejs
- GIT
- Dinosaur
- 공룡게임
- Queue
- class
- dfs
- AWS
- JavaScript
- Express
- 자료구조
- Today
- Total
포시코딩
[Sequelize] FK(Foreign Key) 설정하기 본문
개요
Sequelize를 통해 이미 종속을 진행할 두 테이블을 만들었다는 가정하에 진행한다.
그냥 디비버같은 DBMS 툴에서 설정해줘도 되지만
Sequelize의 생태계를 이해하기 위해 테스트 겸 구현해봤다.
준비
Users 테이블
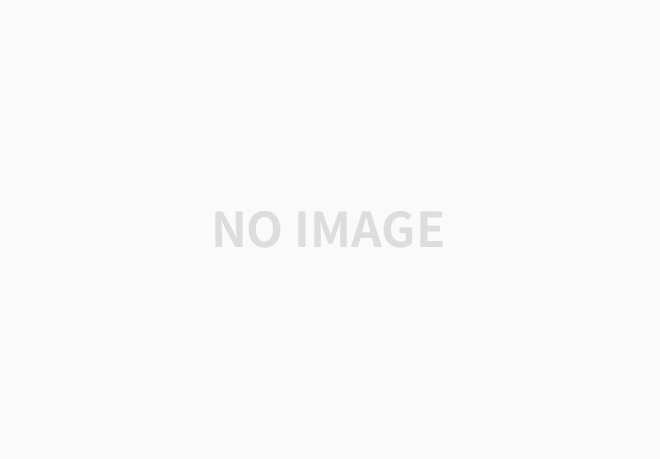
Tokens 테이블
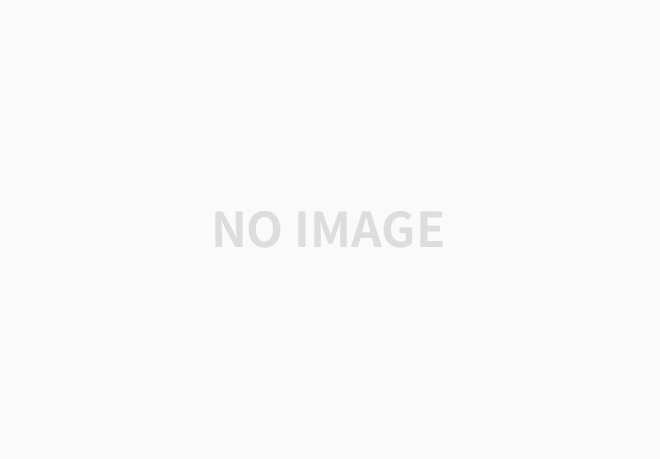
* Users.userId 와 Tokens.userId를 1 대 다 관계로 설정할 것이다.
외래키 설정
npx sequelize migration:generate --name fk-token
먼저, 위 명령어를 통해 외래키 설정을 위한 migration file을 하나 생성해준다.
/migrations 폴더에 어쩌구-fk-token.js 파일이 생겼을것이다. 열어서 기본틀에 아래와 같이 추가로 작성해준다.
'use strict';
/** @type {import('sequelize-cli').Migration} */
module.exports = {
async up (queryInterface, Sequelize) {
/**
* Add altering commands here.
*
* Example:
* await queryInterface.createTable('users', { id: Sequelize.INTEGER });
*/
await queryInterface.addConstraint("Tokens", { // FK를 설정할 테이블 (DB에 있는 테이블 이름과 같아야한다.)
fields: ["userId"], // FK로 등록할 필드 이름
type: "foreign key",
name: "users_tokens_id_fk",
references: {
table: "Users", // 참조할 테이블 (DB에 있는 테이블 이름과 같아야한다.)
field: "userID", // 참조할 필드 이름
},
onDelete: "cascade",
onUpdate: "cascade",
});
},
async down (queryInterface, Sequelize) {
/**
* Add reverting commands here.
*
* Example:
* await queryInterface.dropTable('users');
*/
}
};
다 작성 후 저장한다음 아래 명령어를 통해 DB에 적용시켜준다.
npx sequelize db:migrate
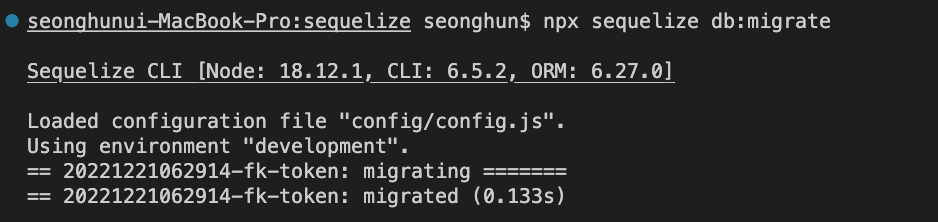
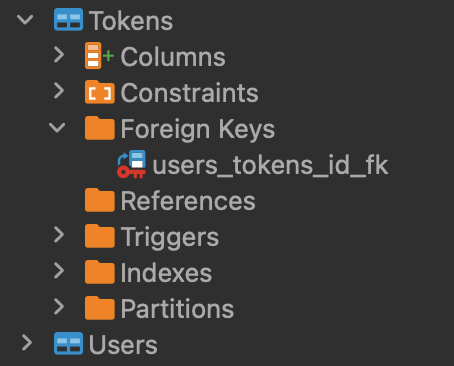
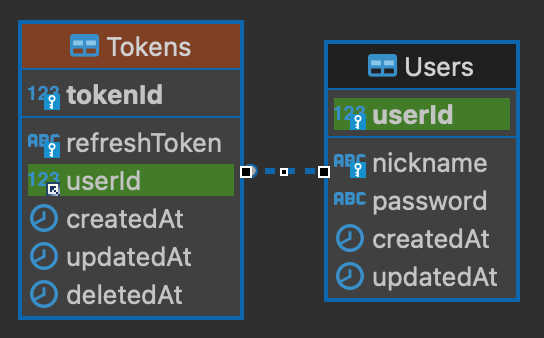
Tokens 테이블의 Foreign Keys에 fk가 잘 들어왔고, 엔티티 관계도에서도 잘 연결되있는걸 볼 수 있다.
Model Mapping
migration을 통해 DB는 변경해줬지만 Model에서의 매핑은 아직 진행하지 않았다.
관계설정을 해야하는데 이해하기 쉽게 나타내면 다음과 같다.
* 1:N 관계 설정
(1 대 다 관계. 유저(1)가 게시글들(N)을 보유한다는 뜻)
hasMany <-> belongsTo
* 1:1 관계 설정
hasOne <-> belongsTo
* N:M 관계 설정
belongsToMany
sequelize/models/user.js
'use strict';
const {
Model
} = require('sequelize');
module.exports = (sequelize, DataTypes) => {
class User extends Model {
/**
* Helper method for defining associations.
* This method is not a part of Sequelize lifecycle.
* The `models/index` file will call this method automatically.
*/
static associate(models) {
// define association here
this.hasMany(models.Token, { foreignKey: "userId" });
}
}
User.init({
userId: {
primaryKey: true,
type: DataTypes.INTEGER,
},
nickname: DataTypes.STRING,
password: DataTypes.STRING
}, {
sequelize,
modelName: 'User',
});
return User;
};
user는 token을 여러개 가질 수 있기 때문에 hasMany를 통해 관계를 지정해준다.
sequelize/models/token.js
'use strict';
const {
Model
} = require('sequelize');
module.exports = (sequelize, DataTypes) => {
class Token extends Model {
/**
* Helper method for defining associations.
* This method is not a part of Sequelize lifecycle.
* The `models/index` file will call this method automatically.
*/
static associate(models) {
// define association here
this.belongsTo(models.User, {foreignKey: 'userId'})
}
}
Token.init({
tokenId: {
primaryKey: true,
type: DataTypes.INTEGER,
},
refreshToken: DataTypes.STRING,
userId: DataTypes.INTEGER
}, {
sequelize,
modelName: 'Token',
});
return Token;
};
token은 user에 종속된다.
위와 같이 진행하게 되면 관계형 Model mapping은 마무리된다.
도움받은 곳
https://loy124.tistory.com/374
Node Sequelize ORM 다루기 - 외래키 생성하기, 관계형 설정하기
loy124.tistory.com/373 Node Sequelize ORM 다루기 - DB 생성및 테이블 생성하기 Sequelieze는 Node에서 사용하는 ORM이다. 여기서 ORM 이란? ORM은 Object Relational Mapping의 약자로써 OOP(Object Oritented Programming)에서 객체
loy124.tistory.com
'Node.js' 카테고리의 다른 글
[Sequelize] migration을 이용한 Table 속성 변경 방법들 (0) | 2022.12.22 |
---|---|
[Sequelize] 이미 생성한 테이블의 컬럼 속성 변경하기 (0) | 2022.12.22 |
[Sequelize] config 파일에서 dotenv를 읽지 못하는 문제 (0) | 2022.12.21 |
[bcrypt] Error: Invalid salt. Salt must be in the form of: $Vers$log2(NumRounds)$saltvalue (0) | 2022.12.21 |
[Sequelize] config.json에 dotenv 환경변수 사용하기 - 작성중 (0) | 2022.12.20 |